Ah! It was in there but it said 4.0 instead of 4.o. Thanks again.
k, now the shaders work fine in vulkan EXCEPT those with the down-rez snippet in it. It turns the screen black with rainbow dots making up the images that are supposed to be there:
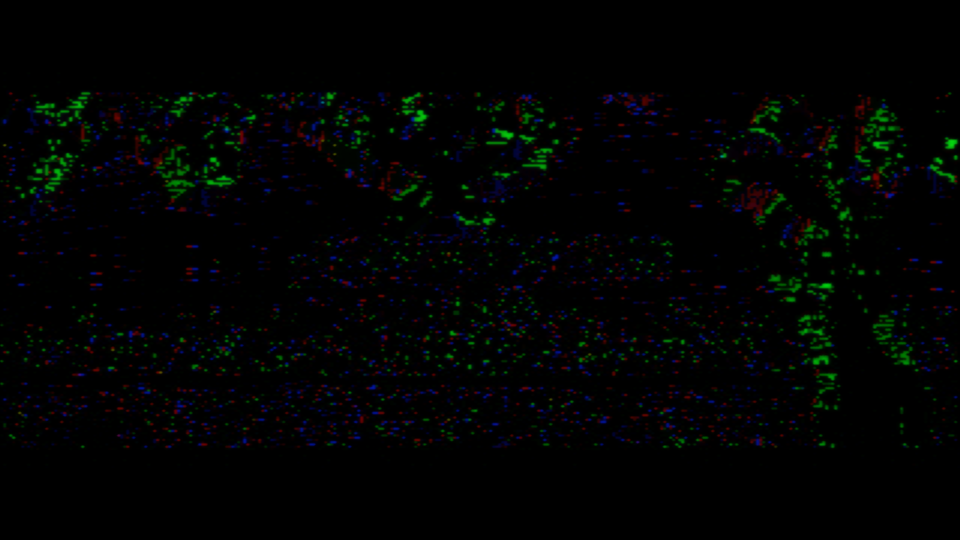
That’s one of the detection passes for scalefx. That means the shader was referring back to a specific pass and putting something in front of them breaks that order.
So what can I do to fix It? It only happens on vulkan and not any other backend.
There are passes that reference “Original” and “PassOutput0”. You would have to add aliases to the preset for the pass before scalefx passes (call it “scalefx_reference” or something like that) and then an alias for the first scalefx pass (“scalefx_pass0” or whatever) and then change those references in the shader files to match.
shaders = 17
shader0 = ./shaders/stock.slang
filter_linear0 = false
scale_type_x0 = absolute
scale_type_y0 = absolute
scale_x0 = 320
scale_y0 = 240
shader1 = ./shaders/scalefx-pass0.slang
filter_linear1 = false
scale_type1 = source
scale1 = 1.0
alias1 = sfxp1
shader2 = ./shaders/scalefx-pass1.slang
filter_linear2 = false
scale_type2 = source
scale2 = 1.0
alias2 = sfxp2
shader3 = ./shaders/scalefx-pass2.slang
filter_linear3 = false
scale_type3 = source
scale3 = 1.0
alias3 = sfxp3
shader4 = ./shaders/scalefx-pass3.slang
filter_linear4 = false
scale_type4 = source
scale4 = 3.0
alias4 = sfxp4
shader5 = ./shaders/scalefx-pass0.slang
filter_linear5 = false
scale_type5 = source
scale5 = 1.0
alias5 = sfxp5
shader6 =./shaders/scalefx-pass1.slang
filter_linear6 = false
scale_type6 = source
scale6 = 1.0
alias6 = sfxp6
shader7 = ./shaders/scalefx-pass2.slang
filter_linear7 = false
scale_type7 = source
scale7 = 1.0
alias7 = sfxp7
shader8 = ./shaders/scalefx-pass7.slang
filter_linear8 = false
scale_type8 = source
scale8 = 3.0
alias8 = sfxp8
shader9 = ./shaders/image-adjustment.slang
filter_linear9 = false
scale_type9 = source
scale9 = 1.0
shader10 = ./shaders/aa-shader-4.o.slang
filter_linear10 = true
scale_type10 = viewport
scale10 = 1.0
shader11 = ./shaders/scanlines.slang
filter_linear11 = false
scale_type11 = source
scale11 = 1.0
alias11 = scanpass
shader12 = ./shaders/bloom-pass-sh1nra358.slang
filter_linear12 = false
scale_type12 = source
scale12 = 1.0
shader13 = ./shaders/blur-gauss-h.slang
filter_linear13 = true
scale_type13 = source
scale13 = 1.0
shader14 = ./shaders/blur-gauss-v.slang
filter_linear14 = true
scale_type14 = source
scale14 = 1.0
shader15 = ./shaders/blur-haze-sh1nra358.slang
filter_linear15 = true
scale_type15 = source
scale15 = 1.0
shader16 = ./shaders/ntsc-colors.slang
filter_linear16 = false
scale_type16 = source
scale15 = 1.0
parameters = "INTERNAL_RES"
INTERNAL_RES = 6.0
indent preformatted text by 4 spaces
Right now, the slangp file looks like this (this is what it looks like by default). Aliases are added to the scalefx portions. What next?
That stock pass needs an alias, as well, and you need to replace all of the instances of “Original” with whatever alias you give it, and you need to replace every instance of PassOutput0 with sfxp1.
Notepad++ did not find anything called ‘original’ or passoutput0 in the stock pass or the scalefx shaders.
You’ll have to change the instances in the actual fragment code, as well.
my version doesn’t look like that because it was converted to vulkan unofficially before it was officially converted into vulkan.
And I liked the look of the old version better than the author’s new version because it is visually identical to 6xbrz and his newest version isn’t:
https://mega.nz/#!758AhKiL!-CxxpDkbzzng45di1CnMF-P7OQt2PCY0oMh8KqMYZQM
That would have been good information to have…
I can only really support our official shader repos, but if you’re using the old scalefx, there’s an “Original” in there, too, so you would need to do the same concept with it.
Im pretty sure it was either you or guest that converted it for me.
scalefx-pass0 #version 450
/*
ScaleFX - Pass 0
by Sp00kyFox, 2016-03-30
Filter: Nearest
Scale: 1x
ScaleFX is an edge interpolation algorithm specialized in pixel art. It was
originally intended as an improvement upon Scale3x but became a new filter in
its own right.
ScaleFX interpolates edges up to level 6 and makes smooth transitions between
different slopes. The filtered picture will only consist of colours present
in the original.
Pass 0 prepares metric data for the next pass.
Copyright (c) 2016 Sp00kyFox - [email protected]
Permission is hereby granted, free of charge, to any person obtaining a copy
of this software and associated documentation files (the "Software"), to deal
in the Software without restriction, including without limitation the rights
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
copies of the Software, and to permit persons to whom the Software is
furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in
all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
THE SOFTWARE.
*/
#pragma name sfxp0
// Reference: http://www.compuphase.com/cmetric.htm
float eq(vec3 A, vec3 B)
{
float r = 0.5 * (A.r + B.r);
vec3 d = A - B;
vec3 c = vec3(2 + r, 4, 3 - r);
return 1 - sqrt(dot(c*d, d)) / 3;
}
layout(set = 0, binding = 0, std140) uniform UBO
{
mat4 MVP;
vec4 SourceSize;
};
#pragma stage vertex
layout(location = 0) in vec4 Position;
layout(location = 1) in vec2 TexCoord;
layout(location = 0) out vec2 vTexCoord;
void main()
{
gl_Position = MVP * Position;
vTexCoord = TexCoord;
}
#pragma stage fragment
layout(location = 0) in vec2 vTexCoord;
layout(location = 0) out vec4 FragColor;
layout(binding = 1) uniform sampler2D Source;
#define TEX(x, y) textureOffset(Source, vTexCoord, ivec2(x, y))
void main()
{
/* grid metric
A B C x y z
E F o w
*/
// read texels
vec3 A = TEX(-1,-1).rgb;
vec3 B = TEX( 0,-1).rgb;
vec3 C = TEX( 1,-1).rgb;
vec3 E = TEX( 0, 0).rgb;
vec3 F = TEX( 1, 0).rgb;
// output
FragColor = vec4(eq(E,A), eq(E,B), eq(E,C), eq(E,F));
}
Nope, no original.
That’s not the pass that has it.
Techincally it is official because after one of you converted it, it was placed in github as the old one. Only the scalefx-pass3 has it. And seems to be only one instance of the word Original. Am I still looking for a PassOutput0 now or no?
Ok, so it’s the old one? That one already has PassOutput0 replaced with the alias, so just the Original is all you need to replace.
so all in all, ive done this:
-
in the pass3 file, i changed layout(binding = 2) uniform sampler2D Original; to layout(binding = 2) uniform sampler2D sfxp3;
-
I added alias0 = sfxp00 to the preset slangp stock file and I added #pragma name sfxp00 to the top of it in the inside.
this is now how the slangp file looks: shaders = 17
shader0 = ./shaders/stock.slang
filter_linear0 = false
scale_type_x0 = absolute
scale_type_y0 = absolute
scale_x0 = 320
scale_y0 = 240
alias0 = sfxp00
shader1 = ./shaders/scalefx-pass0.slang
filter_linear1 = false
scale_type1 = source
scale1 = 1.0
alias1 = sfxp0
shader2 = ./shaders/scalefx-pass1.slang
filter_linear2 = false
scale_type2 = source
scale2 = 1.0
alias2 = sfxp1
shader3 = ./shaders/scalefx-pass2.slang
filter_linear3 = false
scale_type3 = source
scale3 = 1.0
alias3 = sfxp2
shader4 = ./shaders/scalefx-pass3.slang
filter_linear4 = false
scale_type4 = source
scale4 = 3.0
alias4 = sfxp3
shader5 = ./shaders/scalefx-pass0.slang
filter_linear5 = false
scale_type5 = source
scale5 = 1.0
alias5 = sfxp4
shader6 =./shaders/scalefx-pass1.slang
filter_linear6 = false
scale_type6 = source
scale6 = 1.0
alias6 = sfxp5
shader7 = ./shaders/scalefx-pass2.slang
filter_linear7 = false
scale_type7 = source
scale7 = 1.0
alias7 = sfxp6
shader8 = ./shaders/scalefx-pass7.slang
filter_linear8 = false
scale_type8 = source
scale8 = 3.0
alias8 = sfxp7
shader9 = ./shaders/image-adjustment.slang
filter_linear9 = false
scale_type9 = source
scale9 = 1.0
shader10 = ./shaders/aa-shader-4.o.slang
filter_linear10 = true
scale_type10 = viewport
scale10 = 1.0
shader11 = ./shaders/scanlines.slang
filter_linear11 = false
scale_type11 = source
scale11 = 1.0
alias11 = scanpass
shader12 = ./shaders/bloom-pass-sh1nra358.slang
filter_linear12 = false
scale_type12 = source
scale12 = 1.0
shader13 = ./shaders/blur-gauss-h.slang
filter_linear13 = true
scale_type13 = source
scale13 = 1.0
shader14 = ./shaders/blur-gauss-v.slang
filter_linear14 = true
scale_type14 = source
scale14 = 1.0
shader15 = ./shaders/blur-haze-sh1nra358.slang
filter_linear15 = true
scale_type15 = source
scale15 = 1.0
shader16 = ./shaders/ntsc-colors.slang
filter_linear16 = false
scale_type16 = source
scale15 = 1.0
parameters = "INTERNAL_RES"
INTERNAL_RES = 6.0
And now, shaders aren’t loading.
Original is supposed to be replaced with sfxp00. It refers to the shader before the scalefx passes start. There will also be one or more references to Original in the body of the shader. That one line you already changed is just the declaration.
All those times I mentioned that some emu cores crashed with this, this could have been the solution all along
If only you guys incorporated what ppsspp does to use this no matter the rez (while keeping 2D in low res while 3D stays in high rez, that would fix the 3D/2D + high rez issue in retroarch. Thanks again.